How to Build a Redis GUI
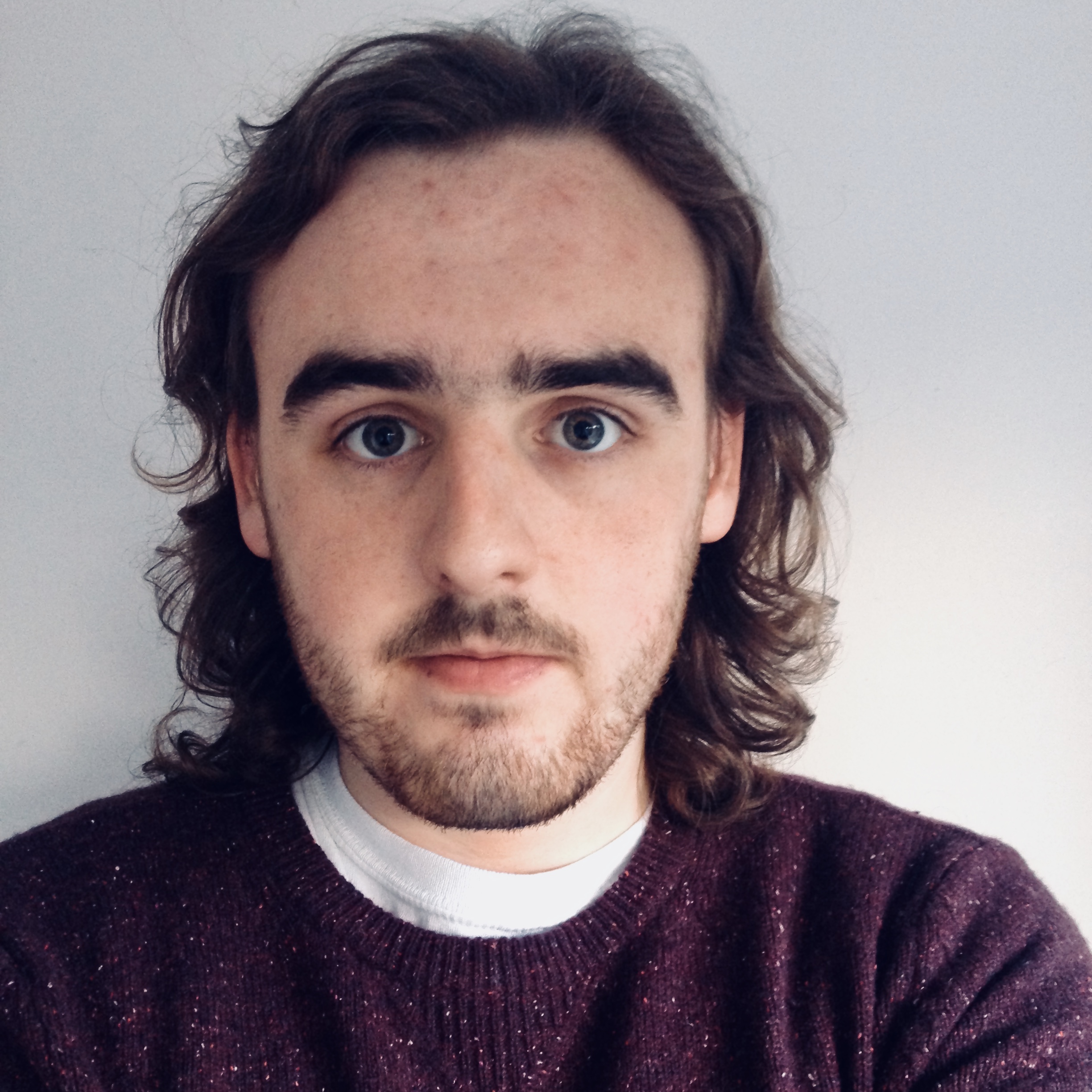
With a Redis GUI, you can make the most out of your database, without memorizing any commands.
Databases are incredible business tools. They allow you to store and manipulate large sets of data. That way, you can make better-informed decisions. They provide you with insights that just wouldn’t be possible with manual data storage.
But they can be quite complex.
Redis is a NoSQL database. It allows you to store data in key/value pairs. It’s a huge shift from the regular SQL paradigm of storing data in tables and rows.
For this reason, Redis is a great tool not just as your main database, but also as an auxiliary data source . You might use a regular DB for most of your data, then use Redis to store sessions, cached data, or really anything you want.
Usually, you can interact with your Redis database using the command line or a programming language. But you can use GUIs (Graphical User Interfaces), to make this process much easier.
With a Redis GUI, you can use a visual interface and just pick the commands you want to execute. In addition, if you want to run your own commands, you can do that too. You can even save custom commands, so you can grow your tool’s functionality over time.
Today you are going to learn how you can build your own Redis GUI in three easy steps. And you don’t even need to know how to code. You can build it using a free low-code builder like Budibase to connect to your database and execute these functions.
Let’s get started!
Which is better: Redis or MongoDB?
Redis is usually slightly faster than MongoDB. But to understand which one is better you need to take into account your requirements.
Redis stores data as key/value pairs, while MongoDB uses documents and collections. MongoDB can handle more complex datasets, and for that, it sacrifices performance.
It’s worth mentioning that although Redis stores data as key/value pairs, it doesn’t mean that it can only store strings. The values of your database entries can be strings, lists, hashes, sets, and other data types.
On that note, we’ve also created a guide to building a MongoDB GUI in Budibase.
Is there a GUI for Redis?
Redis by itself doesn’t have an official GUI. But there are many good options for Redis GUI applications.
You can even build your own Redis GUI by following this tutorial. You can do it in just 3 steps and it requires no coding knowledge.
You might also like our guide to open-source low-code platforms .
How do I visualize a Redis Database?
The easiest way to visualize data is with a Redis GUI. With it, you can display data in any way you want, and get access to commands to manipulate data in many ways.
Today you are going to be a Redis GUI with just one screen. But it has two tabs. The first tab is for premade commands, like this:
This screen contains a form with the command to be executed and two options for how to get the key to be used, with a custom key or picking from a list:
You can use the form to build your command and see the results. In this app, we are using a display design that resembles a command line. But you can customize this design in any way you want to with the Budibase components.
In addition to the premade commands, there’s the “Custom commands” tab. It’s a simple form that allows advanced users to run their own commands:
Check out our in-depth guide to form UI design .
Therefore, you get the best of both worlds. You get quick access to premade commands, but if you want, you can build your own queries as well.
You might also like our guide to building a Firebase GUI .
Now let’s build your app.
Step 1 - Create an app and connect it to your database
If you haven’t already, sign up for Budibase. Then create a new app. If you just want to test out Redis, there’s a free cloud option on their website.
Back to Budibase, on the next screen, pick Redis as your data source and click on continue. Then add your database credentials:
If you have any sort of firewall, don’t forget to whitelist the Budibase IP address.
Once you do this, Budibase can connect with your database.
Next, you can manipulate data using queries. Click on add new query to get this screen:
In it, you can see a few connection types. These are quick commands in case you just want to use one of them. In the demo app, you could really have just one query. But to make this easier to follow along, let’s create two queries in your Redis GUI.
First:
- Query name: command
- Function: Redis command
- Bindings: command (default value ping)
- Fields: {{ command }}
And then:
- Query name: get_keys
- Function: Redis command
- No bindings
- Fields: keys *
- Transformer: return data[0]
The first query is the one you are going to use in your app to load data. After your users fill in the form fields, you send data to this query and display the results.
The second query is used to get a list of the field keys to be used in the dropdown. We use this transformer because a Redis command returns an array by default.
It’s much harder to use this kind of data in components such as options pickers or even tables, repeaters, and cards.
So we transform the default data response from this:
[ [ “firstkey”, “anotherkey”, “newkey” ] ]
Into this:
[ “firstkey”, “anotherkey”, “newkey” ]
That’s all you need on the “Data” tab. Let’s move on to the “Design” tab now.
Step 2 - Create your tabs and forms
Go to Design > Theme. In this section, you can set up what your Redis GUI looks like. Pick the dark theme, and set the button roundness to zero:
You can use round buttons if you want, but with this setting, it’s easier to create your tabs design.
Also, you can add other styling options such as a background color in your header. Click on Navigation, and then add this as the background color:
1radial-gradient(circle farthest-corner at 10% 20%,
2rgba(174,14,87,1) 0%,
3rgba(174,116,12,1) 90% )
You can use not only regular colors but also CSS gradients as well.
On this screen, you can delete the navigation links in case you want a one-page app as we have in our demo.
Now go to screens, add new and pick a blank screen.
This is the elements tree for this portion of the tutorial:
First, add a container component to hold your tabs buttons. Set this container to the horizontal direction, and align left:
With these settings, your buttons are going to be on the same line, and they will be next to each other.
Then add the two buttons to pick which is the active tab. Both have the quiet design selected and this onClick action:
This action saves the current tab. So it’s either Premade or Custom.
Also, make sure that the component name is correct, as we use the name in the tab’s styling.
Now you can add an embed component with this code:
1<style type='text/css'>
2div[data-name="{{ State.tab }} Commands"] >
3button { background: #000 ! important
4</style>
The trick here is to apply a CSS background color only to the tab with the name “[state] Commands”. This is what this embed code does.
Now you need to switch back and forth between the tabs. You can hide and show the tab contents using the display conditions for each of the containers.
The “premade” container has these display conditions:
It’s “hide” if {{ State.tab }} is Custom. And the custom container is the other way around. The display condition there is the “show” if {{ State.tab }} is Custom.
Thus, when one container is visible, the other is hidden at all times.
Both these containers are inside another container. This component is there just to hold the border that goes around the tabs. You can use this CSS code in it:
1border: 5px solid #000;
2margin-top: -34px
And then use some paddings as well if you want. In our demo we have 16px top/bottom and 20px left/right.
Moving on, add a form inside the premade container, this form is there just to hold the form components.
Add an options picker for the “command”. You can manually add the command names you use the most.
If you want a more complex solution, you can get them from a database. This allows you to quickly load most, if not all, Redis commands.
The key type is an options picker with the “radio button” style, in the horizontal mode:
This options picker has two custom options just like the previous one.
Now we want to hide/show the text input or the dropdown for the field keys.
The technique is quite similar to what you used for the tabs. Create containers around each of the options and for the customkey use this display condition:
Hide component if {{ Query.Fields.keytype }} is equal to current
And use the opposite condition on the currentKey container. So make it show the component if the keyType is current.
This ensures that only one of them is visible at a time since they have opposing conditions.
The customkey text field is a simple text field. The current keys picker uses a data provider with the get_keys query:
Then, on the options picker, select the data provider as your options source:
You can add a text field for additional parameters, and also a text field for the custom query. They are simple text fields.
The real magic happens on the “Run query” button.
There are two buttons, one for each tab. Both of them do the same thing. The run button saves the current form fields as a “query” appState.
Here is an example:
In the premade tab, you can use this JS code:
1var field = $("Query.Fields.keytype");
2if ( field && "custom" == field) {
3field = $("Query.Fields.customkey");
4} else {
5field = $("Query.Fields.currentkeys");
6}
7return $("Query.Fields.command") +
8" " + field + " " + $("Query.Fields.more")
This code snippet saves the command, field, and the additional parameters as a single text line in the “query” appState.
That’s what allows your Redis GUI to load commands as your users pick them.
You’ll use a very similar approach to the custom query tab. The “run” button in this case simply saves the “query” appstate with this value:
{{ CustomQuery.Fields.fullcommand }}
Therefore, the “query” appState is just a mirror of the value in the text field for the custom query tab.
Now let’s process and display this data.
Step 3 - Display your Redis GUI data
There are just a couple of components left to build your Redis GUI. This is the components tree for that section of the page:
There are two markdown components.
The first one contains the query command. It is that stylized command that looks like a command prompt with a > sign and a different color for the first word.
As you might have guessed, this component reads data from the query appState.
To make it look like a command line, add this Custom CSS to it:
font-family: Monaco, Consolas, ‘Courier New’, ‘Courier’, monospace
Then on the markdown contents use this JS function:
1var query = $("State.query");
2if ( ! query ) {
3query = "ping";
4}
5query = query.split(' ');
6query[0] = "<div style='background:
7rgba(255, 255, 255, 0.1);
8padding: 5px 10px'>
9<strong style='color: rgb(234 179 8)'>>
10</strong>
11<strong style='color: rgb(147, 45, 250)'>"
12
13query[0] + " </strong> <strong> ";
14last = query.length - 1;
15query[last] = query[last] + "</strong></div>";
16query = query.join(" ");
17return query
This function checks if the query appState is set. If it isn’t, it sets it with the default state (ping). Then, it breaks the command down into two parts, the first one gets a different color, and the second part is bold.
The results data provider is calling your generic “command” query. You can pass the query appState as a binding:
To make sure that the default data is correctly used, you can use this JS function:
1if ( $("State.query") ) {
2return $("State.query");
3} else {
4return "ping"
5}
So it either returns the query appState or a ping command.
The data itself is all extracted into a paragraph. You can use this JS function in it:
1var data = $("results.Rows");
2if ( data && data.length > 0 ) {
3data = JSON.stringify(data, null, '\t')
4} else {
5data =
6"no data - check if your command is correct";
7}
8return data;
This code checks if there are valid results. If there are, use the stringify function to display the variables nicely. If there is no data, display an error message.
How to build a Redis GUI with Budibase
Today you learned how you can create a Redis GUI app without coding. You learned some basic Redis database interactions, but also advanced settings in Budibase. These steps can be used to build any GUI or app you want.
The sky is the limit now. You can improve your app by saving command lines, allowing multiple commands, and adding logs.
Check out our ultimate guide to building a database GUI in Budibaes for more inspiration.
We hope you enjoyed it, and see you again next time!